5 min to read
Reactive Programming with Signals
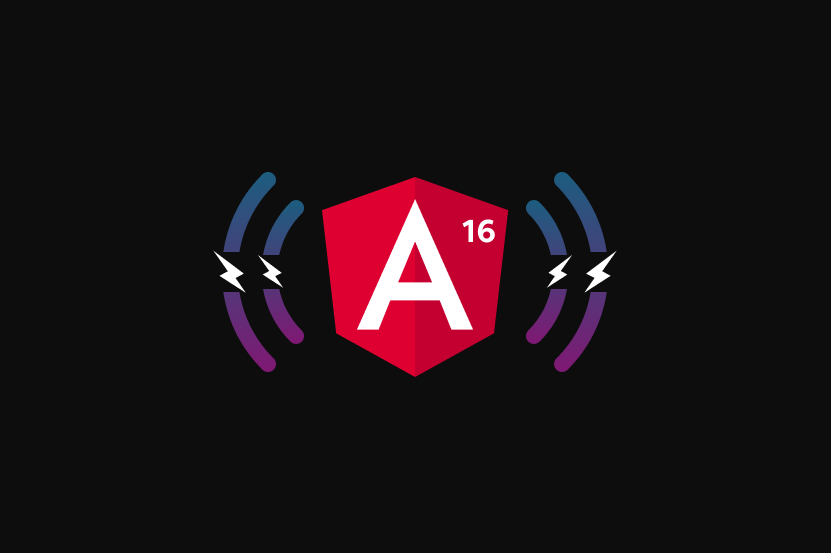
Reactive Programming with Signals
How are you doing? Today’s post is dedicated to reactive programming in Angular using Signals, where we will be reviewing concepts, techniques needed to implement signals, integration and compatibility with RxJS, advantages and exercises; as always the official docu here
What are Signals in Angular??
With Angular 16, a new way of handling reactivity is introduced: Signals. These allow us to manage state more efficiently and easily, without needing to rely on Observables and subscriptions.
The Signals were introduced in Angular 16 as a simpler and more efficient alternative for handling reactivity. In previous versions of Angular, reactivity handling was centered around Observables and RxJS, which in some situations, could be complex and generate more cumbersome code. The Signals seek to simplify this, offering another, more direct, way to interact with reactive data (whether primitive values or data structures), avoiding also, the complexities that could involve subscriptions and the lifecycle of Observables.
How do Signals work?
Signals** allow you to store and manage reagent values. Unlike Observables, no manual subscriptions are required. The **Signals** manage reactivity automatically. When a value changes, Angular updates the view automatically.
To create a Signal, simply use the signal()
function by passing an initial value.
import { signal } from "@angular/core";
export class CounterComponent {
// Crear un Signal con valor inicial
counter = signal(0);
// Incrementamos el valor del contador
increment() {
this.counter.set(this.counter() + 1);
}
}
In this example:
counter
is a Signal that has an initial value of0
.- We use
counter()
to get the value of the Signal. - To update the value of
counter
, we usecounter.set()
.
A computed Signal depends on one or more Signals. When the Signals on which it depends change, the Computed Signal is automatically recalculated.
import { signal, computed } from "@angular/core";
export class CounterComponent {
counter = signal(0);
// Signal Computado que depende de counter
message = computed(() => {
return `The counter value is: ${this.counter()}`;
});
increment() {
this.counter.set(this.counter() + 1);
}
}
In this example:
message
is a Computed Signal that depends on the Signalcounter
.- Each time
counter
changes,message
is automatically recalculated. - We cannot update
message
directly, since it is a Computed Signal (read-only) and its value is derived fromcounter
(it is recalculated based on changes in the value of counter).
Advantages of Signals.
- Simplicity: Signals are simpler to implement than Observables. No need to worry about subscriptions, cancellations or complex combinations of streams.
- Performance: Being more direct, Signals are faster and lighter compared to Observables when reactivity is simple.
- Code reduction: Signals require less code, which makes our applications more readable, scalable and maintainable.
- Automatic reactivity: Changes to a Signal automatically update the view, which simplifies UI management.
When to use Signals?
Signals are ideal when we need to handle simple state values reactively, without the need for subscriptions or advanced manipulation of streams or data streams, for example:
- counters,
- boolean values,
- simple data structures that do not require mappings.
It is not recommended to use Signals when we are working with complex applications that require advanced reactivity, such as complex data combinations, handling multiple streams, transformations and data mappings.
Bonus: Integration of Signals and RxJS in Angular.
In Angular, we can easily combine the reactivity of Signals with the power of RxJS, one example being, using two key methods: toSignal
and toObservable
. These methods facilitate interoperability between the two reactive systems, allowing us to take advantage of the best of both worlds.
Create a Signal from an Observable with toSignal:
The toSignal
method allows us to convert an RxJS** Observable into an Angular Signal. This is useful for example, when we want to use an existing Observable and handle its value reactively with Signals, without losing the ability to get automatic updates in the UI.
Create an Observable from a Signal with toObservable:
On the other hand, toObservable
allows us to convert a Signal into an Observable. This is useful if we need, for example, a Signal to interact with other data streams in our app that use RxJS, allowing Signals to be integrated into an ecosystem based on Observables.
Over here I’m passing the official docu with more details about Signals and RxJS integration
Conclusion
The Signals offer us a more direct and simple way to manage reactivity in Angular. By removing the complexity of Observables, Signals become an ideal tool for projects and functionalities with simplified and optimized state logic.
For more complex applications, where we need to handle multiple data streams and advanced transformations, RxJS and Observables remain the best choice.
Happy coding!