4 min to read
SASS
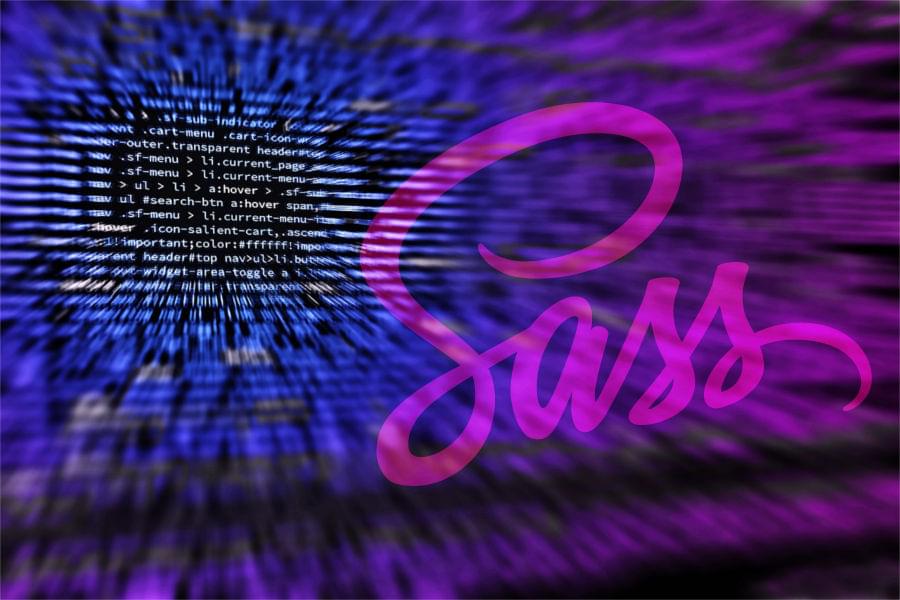
SASS: Tricks & Tips (or treat)
Hello, how are you? A few days after Halloween, today’s post is dedicated to SASS!!! Some tricks, dark magics and little tips that if you didn’t know them, they can be very useful when working with this magic!
Interpolation
To access values using interpolation:
$white: #ffffffff;
/**
Color Reference
Walter is: #{$white}
**/
Placeholders
In order to extend and reuse certain rules in our placeholders, we can define the following:
%color-test-a {
background-color: $prussian;
color: $crimson;
}
%color-test-b {
background-color: $coral;
color: $perano;
}
Once we have defined the placeholders, we can extend them wherever we like. For that, we are going to simulate that we have a container to apply the first placeholder and an alteration of the container (modifier) for the second placeholder. Assuming for this example that the HTML classes are container and container–secondary. Let’s see how it looks like!
.container {
@extend %color-test-a;
&--secondary {
@extend %color-test-b;
}
}
when we compile, we will be left with this result (the colors are up to you, with fries and ketchup would be fine)
.container {
background-color: #B2CBF5;
color: #F2CBF5;
}
.container--secondary {
background-color: #D2CBFF5;
color: #G2CBFF5;
}
If you do A/B testing, it can be useful to have this kind of modifiers set up like this! Note: in my case I use NameThatColor for the names!
Suffixes
If you are working with BEM, it may not be new to you, but a neat and organized way to create suffixes for our classes is to simply use “&-“ or “&__” to create something like this:
.container {
&-pink {}
&__wrapper {}
}
compiled, these selectors would look like this:
.container-pink {}
.container__wrapper {}
Mixins
Last time I wrote a lot about mixins, so I leave a little detail for when we want to add a default value to our parameterizable mixin:
@mixin alert-text($color: #f33) {
color: $color;
}
This way, we are always going to have a default color, so that when we invoke the mixin, we can have the option to send it by parameter another color, or not send it anything so that it uses the one we defined, like this:
@include alert-text();
@include alert-text($black);
Note: In the example we see param color, but we can pass what we define in each mixin, size, margin, width, blah blah and dale que vaaaa
Null
Another interesting variant speaking of parameters by default, is when we use null. If we apply it, the style directly will not be applied unless we really need it, it is good to save us rules that we do not want that they are compiled. Let’s see!
@mixin holis($opacity: null) {
color: $white;
opacity: $opacity;
}
When we use it with param:
.container {
@include holis(0.75);
}
it will compile us:
.container {
color: #ffffffff;
opacity: 0.75;
}
Nowaaa if we don’t pass orange:
.container {
@include holis();
}
it will compile us like this:
.container {
color: #ffffffff;
}
Pretty cool, isn’t it?
@debug
Well, as JS lovers, we have and use console.log().
Here we have something similar! Sometimes we will want to know the result of a function or simply the result of an expression for example:
@debug saturation(#e1d7d2);
@debug saturation(#e1d7d2) > 50;
It will show us respectively:
20%
false
Note: If you want to see other available functions here
Bonus: @for for own classes
If you want to do something more fancy like creating your own bootstrap classes, toppp VIP jewel, or if you are in a re-branding project and also use atomic classes, you can use it! Using @for you can build something like this (and more similar ones you can find everywhere):
@for $i from 1 through 10 {
.p-b-#{$i * 5} {
padding-bottom: #{$i * 5}unquote(px);
}
}
That will generate the following:
.p-b-5 {
padding-bottom: 5px;
}
.p-b-10 {
padding-bottom: 10px;
}
.p-b-15 {
padding-bottom: 15px;
}
.p-b-20 {
padding-bottom: 20px;
}
and the list goes on… (up to 10 in this case). But the idea is to be able to generate dynamic classes with the values that we calculate, not only for paddings or margins but for whatever you can think of that can be useful in each case!
Happy cooooooding!